Computação Gráfica: Filtros e Processamento de Imagens Digitais
De Aulas
Revisão de 16h04min de 5 de abril de 2022 por Admin (discussão | contribs) (Criou página com 'Afluentes: Computação Gráfica = Filtros = Iremos usar para os exemplos a mesma imagem utilizada no conteúdo anterior. <center>200px</cent...')
Afluentes: Computação Gráfica
Filtros
Iremos usar para os exemplos a mesma imagem utilizada no conteúdo anterior.
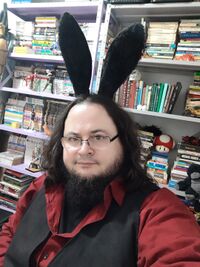
Tons de Cinza
1import sys
2import pygame
3
4
5def gray_scale(img):
6 for y in range(image.get_height()):
7 for x in range(image.get_width()):
8 r, g, b, a = image.get_at((x, y))
9 c = ((0.299 * r) + (0.587 * g) + (0.114 * b))
10 image.set_at((x, y), (c, c, c))
11
12
13argc = len(sys.argv)
14img_in = sys.argv[1]
15img_out = sys.argv[2]
16
17pygame.init()
18
19image = pygame.image.load(img_in)
20w = image.get_width()
21h = image.get_height()
22display_surface = pygame.display.set_mode((w, h))
23
24gray_scale(image)
25
26pygame.image.save(image, img_out)
27
28pygame.display.set_caption(img_in + ' -> ' + img_out)
29
30finish = False
31while not finish:
32 display_surface.blit(image, (0, 0))
33 for event in pygame.event.get():
34 if event.type == pygame.QUIT:
35 finish = True
36 elif event.type == pygame.KEYDOWN:
37 if event.key == pygame.K_ESCAPE:
38 finish = True
39 pygame.display.update()
40pygame.quit()
41quit()
Lineart
1import sys
2import pygame
3
4
5def line_art(image, surface, limiar):
6 surface.fill((255, 255, 255))
7 for y in range(image.get_height()):
8 for x in range(image.get_width()):
9 # primeiro calculamos o tom de cinza do pixel
10 r, g, b, a = image.get_at((x, y))
11 c = ((0.299 * r) + (0.587 * g) + (0.114 * b))
12 # e depois separamos pelo limiar
13 if c < limiar:
14 c = 0 # se menor que o limiar, fica preto
15 else:
16 c = 255 # se maior, fica branco
17 surface.set_at((x, y), (c, c, c))
18
19
20argc = len(sys.argv)
21img_in = sys.argv[1]
22img_out = sys.argv[2]
23limiar = 100
24
25if len(sys.argv) == 4:
26 limiar = int(sys.argv[3])
27
28pygame.init()
29
30image = pygame.image.load(img_in)
31w = image.get_width()
32h = image.get_height()
33surface = pygame.display.set_mode((w, h))
34
35line_art(image, surface, limiar)
36
37pygame.display.set_caption(
38 img_in + ' -> ' + img_out + ' limiar: ' + str(limiar))
39
40finish = False
41pygame.key.set_repeat(1)
42while not finish:
43 for event in pygame.event.get():
44 keypressed = False
45 if event.type == pygame.QUIT:
46 finish = True
47 elif event.type == pygame.KEYDOWN:
48 # Vamos as teclas para mudar o limiar em
49 # tempo de execução. Seta para cima aumenta
50 # o limiar e seta para baixo diminui
51 if event.key == pygame.K_ESCAPE:
52 finish = True
53 if event.key == pygame.K_UP:
54 limiar += 1
55 keypressed = True
56 if limiar > 255:
57 limiar = 255
58 if event.key == pygame.K_DOWN:
59 limiar -= 1
60 keypressed = True
61 if limiar < 0:
62 limiar = 0
63 if keypressed:
64 print('limar:', limiar)
65 line_art(image, surface, limiar)
66 pygame.display.update()
67
68pygame.image.save(surface, img_out)
69
70pygame.quit()
71quit()
Detecção de Bordas Roberts
1import sys
2import pygame
3
4
5def gray_scale(img):
6 for y in range(image.get_height()):
7 for x in range(image.get_width()):
8 r, g, b, a = image.get_at((x, y))
9 c = ((0.299 * r) + (0.587 * g) + (0.114 * b))
10 image.set_at((x, y), (c, c, c))
11
12'''
13Matrixes de convolução do algoritmo de Roberts para detecção
14de bordas
15
16GX = | +1 0 |
17 | 0 -1 |
18
19GY = | 0 +1 |
20 | -1 0 |
21'''
22
23
24def roberts(img):
25 for y in range(h-1):
26 for x in range(w-1):
27 a, _, _, _ = image.get_at((x, y))
28 b, _, _, _ = image.get_at((x+1, y))
29 c, _, _, _ = image.get_at((x, y+1))
30 d, _, _, _ = image.get_at((x+1, y+1))
31
32 gx = d - a
33 gy = b - c
34 g = abs(abs(gx) + abs(gy))
35 if g > 255:
36 g = 255
37 image.set_at((x, y), (g, g, g))
38
39
40argc = len(sys.argv)
41img_in = sys.argv[1]
42img_out = sys.argv[2]
43
44pygame.init()
45white = (255, 255, 255)
46image = pygame.image.load(img_in)
47w = image.get_width()
48h = image.get_height()
49display_surface = pygame.display.set_mode((w, h))
50
51gray_scale(image) # Primeiro deixamos em tons de cinza
52roberts(image) # Depois detectamos as bordas usando Roberts
53
54pygame.image.save(image, img_out)
55
56pygame.display.set_caption("Roberts - " + img_out)
57
58finish = False
59while not finish:
60 for event in pygame.event.get():
61 if event.type == pygame.QUIT:
62 finish = True
63 elif event.type == pygame.KEYDOWN:
64 if event.key == pygame.K_ESCAPE:
65 finish = True
66 display_surface.blit(image, (0, 0))
67 pygame.display.update()
68pygame.quit()
69quit()
Histograma de Frequência
O histograma de uma imagem é simplesmente um conjunto de números indicando o percentual de pixels naquela imagem que apresentam um determinado nível de cinza. Estes valores são normalmente representados por um gráfico de barras que fornece para cada nível de cinza o número (ou o percentual) de pixels correspondentes na imagem. Através da visualização do histograma de uma imagem obtemos uma indicação de sua qualidade quanto ao nível de contraste e quanto ao seu brilho médio (se a imagem é predominantemente clara ou escura).
1import sys
2import pygame
3import matplotlib.pyplot as plt
4
5pygame.init()
6
7filename = sys.argv[1]
8image = pygame.image.load(filename)
9
10hist_x = []
11hist_y = []
12for i in range(256):
13 hist_x.append(i)
14 hist_y.append(0)
15
16for y in range(image.get_height()):
17 for x in range(image.get_width()):
18 r, g, b, a = image.get_at((x, y))
19 gray = int((0.299 * r) + (0.587 * g) + (0.114 * b))
20 hist_y[gray] += 1
21
22plt.plot(hist_x, hist_y)
23plt.show()
24
25pygame.quit()
26quit()
Histograma de Frequência de Imagens Coloridas
1import sys
2import pygame
3import matplotlib.pyplot as plt
4
5pygame.init()
6
7filename = sys.argv[1]
8image = pygame.image.load(filename)
9
10hist_x = []
11hist_yr = []
12hist_yg = []
13hist_yb = []
14for i in range(256):
15 hist_x.append(i)
16 hist_yr.append(0)
17 hist_yg.append(0)
18 hist_yb.append(0)
19
20for y in range(image.get_height()):
21 for x in range(image.get_width()):
22 r, g, b, a = image.get_at((x, y))
23 hist_yr[r] += 1
24 hist_yg[g] += 1
25 hist_yb[b] += 1
26
27plt.plot(hist_x, hist_yr, color="red")
28plt.plot(hist_x, hist_yg, color="green")
29plt.plot(hist_x, hist_yb, color="blue")
30plt.show()
31
32pygame.quit()
33quit()