Mudanças entre as edições de "Computação Gráfica: Transformações em Imagens Matriciais"
De Aulas
Linha 84: | Linha 84: | ||
O exemplo aumenta a imagem em 20% da original (imagem.jpg), criando uma imagem resultado chamada out.jpg. | O exemplo aumenta a imagem em 20% da original (imagem.jpg), criando uma imagem resultado chamada out.jpg. | ||
+ | |||
+ | = Espelhamento = | ||
+ | |||
+ | <syntaxhighlight lang=python line> | ||
+ | import sys | ||
+ | import pygame | ||
+ | |||
+ | # Função de espelhamento. O resultado é colocado em surface | ||
+ | def flip(image, surface): | ||
+ | w = image.get_width() | ||
+ | h = image.get_height() | ||
+ | for y in range(h): | ||
+ | for x in range(w): | ||
+ | # coloca o último pixel na primeira posição, | ||
+ | # o penúltimo na segunda, e assim por diante. | ||
+ | surface.set_at((w - 1 - x, y), image.get_at((x, y))) | ||
+ | |||
+ | |||
+ | # PROGRAMA PRINCIPAL | ||
+ | pygame.init() | ||
+ | |||
+ | file_in = sys.argv[1] | ||
+ | file_out = sys.argv[2] | ||
+ | |||
+ | image = pygame.image.load(file_in) | ||
+ | w = image.get_width() | ||
+ | h = image.get_height() | ||
+ | |||
+ | # cria a surface com a proporção a ser alterada | ||
+ | surface = pygame.display.set_mode((w, h)) | ||
+ | |||
+ | # chama a função fazer o espelhamento | ||
+ | flip(image, surface) | ||
+ | |||
+ | # salva a imagem | ||
+ | pygame.image.save(surface, file_out) | ||
+ | |||
+ | pygame.display.set_caption(file_in) | ||
+ | |||
+ | finish = False | ||
+ | while not finish: | ||
+ | for event in pygame.event.get(): | ||
+ | if event.type == pygame.QUIT: | ||
+ | finish = True | ||
+ | elif event.type == pygame.KEYDOWN: | ||
+ | if event.key == pygame.K_ESCAPE: | ||
+ | finish = True | ||
+ | pygame.display.update() | ||
+ | pygame.quit() | ||
+ | quit() | ||
+ | </syntaxhighlight> |
Edição das 11h07min de 16 de março de 2022
Afluentes: Computação Gráfica
Transformações Geométricas
As transformações geométricas são operações de processamento de imagem para alterar a posição inicial dos seus píxels. Dentre algumas operações, temos ampliação, diminuição, espelhamento, rotação, distorção, etc.. Veremos alguns deles aqui.
Para nossos testes, iremos utilizar a imagem abaixo:
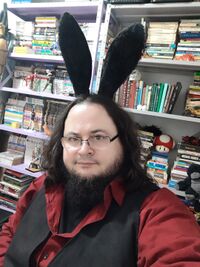
Alteração de Dimensões
1import sys
2import pygame
3
4
5# Retorna x e y equivalente da imagem original na destino
6def get_dest_xy(x_orig, y_orig, proportion):
7 x = int(proportion * x_orig / 100) # calcula a regra de tres de x
8 y = int(proportion * y_orig / 100) # calcula a regra de tres de y
9 return x, y
10
11
12# Retorna x e y equivalente da imagem destino na original
13def get_orig_xy(x_dest, y_dest, proportion):
14 p = 100 / (proportion / 100) # pega o percentual invertido
15 x = int(p * x_dest / 100) # calcula a regra de tres de x
16 y = int(p * y_dest / 100) # calcula a regra de tres de y
17 return x, y
18
19
20# Redimensiona a imagem
21def resize(image, surface, proportion):
22 # Para cada coluna da imagem de destino
23 for y in range(surface.get_height()):
24 # Para cada linha da imagem de destino
25 for x in range(surface.get_width()):
26 # pega o x e y relativo da imagem original
27 x2, y2 = get_orig_xy(x, y, proportion)
28 # e pinta na imagem de destino
29 surface.set_at((x, y), image.get_at((x2, y2)))
30
31
32# PROGRAMA PRINCIPAL
33pygame.init()
34
35file_in = sys.argv[1]
36file_out = sys.argv[2]
37proportion = int(sys.argv[3])
38
39image = pygame.image.load(file_in)
40w = image.get_width()
41h = image.get_height()
42sw, sh = get_dest_xy(w, h, proportion)
43
44# cria a surface com a proporção a ser alterada
45surface = pygame.display.set_mode((sw, sh))
46
47# chama a função pra redimensionar
48resize(image, surface, proportion)
49
50# salva a surface como nova imagem
51pygame.image.save(surface, file_out)
52
53pygame.display.set_caption(file_in)
54
55finish = False
56while not finish:
57 for event in pygame.event.get():
58 if event.type == pygame.QUIT:
59 finish = True
60 elif event.type == pygame.KEYDOWN:
61 if event.key == pygame.K_ESCAPE:
62 finish = True
63 pygame.display.update()
64pygame.quit()
65quit()
- Exemplo de execução
python3.9 resize.py imagem.jpg out.jpg 120
O exemplo aumenta a imagem em 20% da original (imagem.jpg), criando uma imagem resultado chamada out.jpg.
Espelhamento
1import sys
2import pygame
3
4# Função de espelhamento. O resultado é colocado em surface
5def flip(image, surface):
6 w = image.get_width()
7 h = image.get_height()
8 for y in range(h):
9 for x in range(w):
10 # coloca o último pixel na primeira posição,
11 # o penúltimo na segunda, e assim por diante.
12 surface.set_at((w - 1 - x, y), image.get_at((x, y)))
13
14
15# PROGRAMA PRINCIPAL
16pygame.init()
17
18file_in = sys.argv[1]
19file_out = sys.argv[2]
20
21image = pygame.image.load(file_in)
22w = image.get_width()
23h = image.get_height()
24
25# cria a surface com a proporção a ser alterada
26surface = pygame.display.set_mode((w, h))
27
28# chama a função fazer o espelhamento
29flip(image, surface)
30
31# salva a imagem
32pygame.image.save(surface, file_out)
33
34pygame.display.set_caption(file_in)
35
36finish = False
37while not finish:
38 for event in pygame.event.get():
39 if event.type == pygame.QUIT:
40 finish = True
41 elif event.type == pygame.KEYDOWN:
42 if event.key == pygame.K_ESCAPE:
43 finish = True
44 pygame.display.update()
45pygame.quit()
46quit()